Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Python Libraries for Robinhood API Integration and Technical Analysis
Python offers several libraries to bridge the gap between your code and the Robinhood API, making it possible to build automated trading systems and delve deeper into technical analysis. The `robinstocks` library is a popular choice for interacting with the Robinhood API. It simplifies tasks like placing trades and handling user authentication, essentially laying the groundwork for creating automated trading strategies.
However, simply placing trades isn't enough for sophisticated strategies. The `ta` library, specialized in technical analysis, provides a wide range of indicators to extract insights from financial data. These insights are vital for crafting and refining trading algorithms.
Together, these tools provide the core functionality to build Python-based trading bots that can access and use Robinhood's features. Features like accessing real-time data, retrieving tax documents, and even potentially integrating other services (like Twitter for sentiment analysis) expand the potential of these bots. Yet, it's crucial to acknowledge the limitations of these tools and carefully consider the implications of automated trading before implementing complex strategies. While they offer a powerful avenue for exploring trading automation, users should remain aware of the intricacies of the Robinhood API and the broader financial landscape.
The Robinhood API, accessible since 2012, offers a way for developers to build applications that interact with the Robinhood platform. However, it's crucial to remember that it's an unofficial API, meaning Robinhood doesn't officially support it. This can lead to unforeseen issues if Robinhood makes changes that break your integration. The `robinstocks` library stands out as a popular choice for managing this interaction, allowing Python-based trade execution. It's a good example of the open-source projects that have sprung up around the Robinhood ecosystem, giving us access to a range of features beyond just trading, including historical data retrieval.
This Robinhood API opens the door to trading in a variety of asset classes including stocks, options, ETFs, and cryptocurrencies, offering real-time updates on things like ticker prices and portfolio performance. But, it has a limited set of built-in technical indicators, potentially hindering traders who depend on a wider range of indicators to make their decisions. We'll likely need external Python libraries like `ta` or others to build more robust analytical capabilities. Further, because of rate limits, it's important to design your bot carefully so it doesn't trigger a shutdown through excessive API requests. This usually means planning your code to efficiently manage API interactions.
Log-in to the Robinhood API involves retrieving a token, allowing for ongoing user sessions. The `robinstocks` library further enables you to create automated trading strategies – your own “robo-investor,” if you will. Running a Jupyter Notebook, a web-based development environment, tends to be the most efficient way to manage these activities. We essentially get a Python wrapper that connects us to functionality like real-time data on stocks or evaluation of your portfolio performance. Some nice-to-haves within the Robinhood API wrappers are features related to tax document retrieval or dividend tracking. While not strictly necessary, the ability to incorporate features like sentiment analysis through Twitter API keys adds flexibility. This is a good example of the modularity you can gain through Python's open-source library landscape.
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Setting Up a Trading Bot in Under 10 Minutes Using Jupyter Notebook
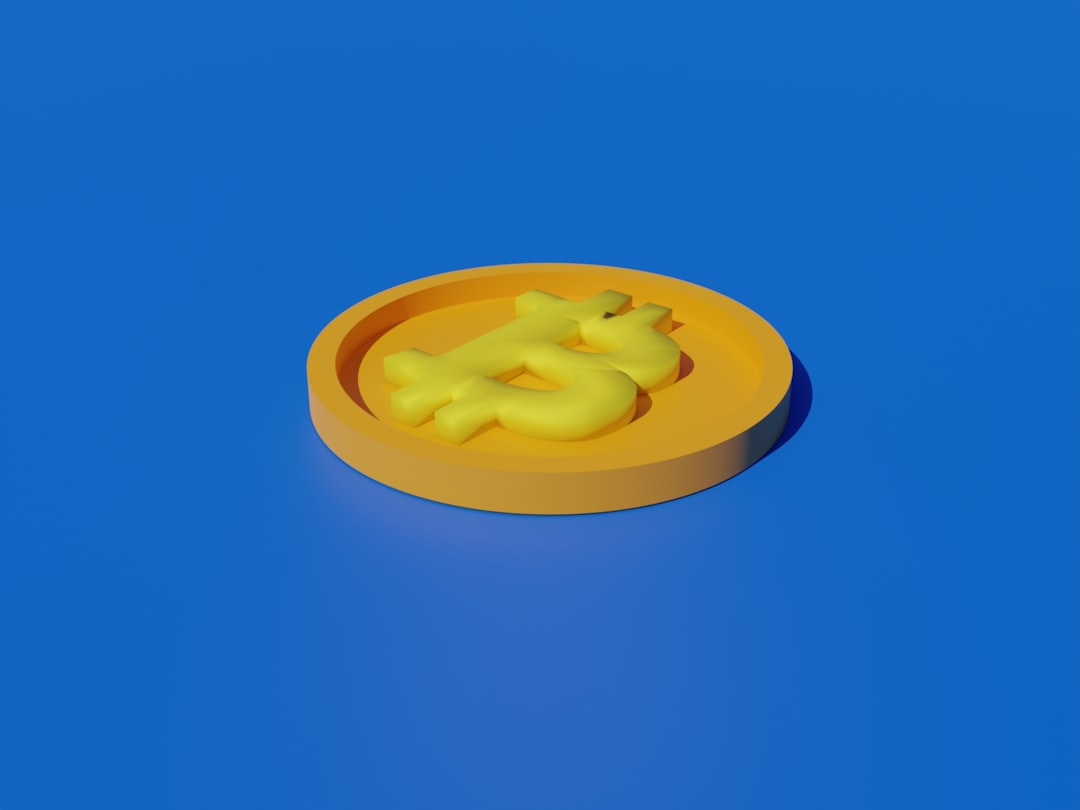
Creating a basic trading bot within Jupyter Notebook can be surprisingly fast, often taking less than ten minutes. The process starts with a prepared Python environment and obtaining necessary API keys from a brokerage like Robinhood or a similar platform. Python libraries like `pandas` for data wrangling and `matplotlib` for visualizations are readily available to assist in building trading strategies. Jupyter Notebook's interactive environment makes it particularly useful for sketching out and testing your initial trading ideas. While quick to set up, it's strongly recommended to initially use a "Paper Trading" mode to experiment and refine strategies before deploying them with real money. This streamlined approach can be a valuable introduction to algorithmic trading, but it's important to be mindful of the potential downsides and limitations inherent in any automated trading system.
Setting up a trading bot quickly using Jupyter Notebook can be surprisingly straightforward. The interactive nature of Jupyter, with its immediate feedback loop on code execution, allows for rapid prototyping – a basic bot can be built within 10 minutes. This rapid development cycle can be especially beneficial when experimenting with various strategies.
Having an environment where you can quickly manipulate real-time market data from the Robinhood API is key for building bots that react to sudden market shifts. This responsiveness is a must for trading strategies that rely on high-frequency adjustments.
Moreover, the Jupyter Notebook format provides a clean way to organize and document your code. This can be quite handy if you're working in a team or contributing to a broader coding community where clarity and readability are important. Jupyter has some built-in features for data visualization that are directly integrated into the notebook environment through libraries like Matplotlib and Seaborn. The advantage here is that you're able to see visual representations of your trading results right next to the code generating them, which is a nice way to analyze trends and outcomes.
Further, if your trading strategy involves more complex calculations, Jupyter offers the flexibility to swap out different programming languages, called kernels, if needed. This means you could potentially use languages like R or Julia alongside Python to adapt the computational methods to the needs of the trading logic. While it's less formal, this ability to quickly test various approaches is one of the benefits of working in a Jupyter environment.
Jupyter's cell-based structure comes in handy for debugging as well. When you're trying to pinpoint the source of an error, you can isolate code sections, running and testing parts independently. This iterative process of debugging and code refinement can become more efficient with a tool like Jupyter.
Version control is another benefit when using Jupyter. The fact that notebooks can be saved in a structured format like JSON makes it fairly easy to use common version control tools like Git. This is useful when collaborating with others or needing a clear history of code changes during development.
One of the things Jupyter does well is facilitate informal testing. You don't have to set up a large, complex infrastructure to experiment with your bot using historical data. This ability to test things quickly helps accelerate the development process without the overhead of creating a full-fledged, enterprise-level environment.
There's also potential to incorporate some machine learning into your trading strategies. Scikit-Learn, a popular Python library, can be used within Jupyter, giving you the chance to build predictive models that go beyond basic technical indicators to potentially forecast future market trends.
Jupyter has a strong community surrounding it. This can be helpful when you're encountering issues as there are many online resources such as tutorials and forums to assist with problem-solving. You can benefit from other people's experiences and potentially learn from the pitfalls they've encountered along the way.
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Implementing Multi-Factor Authentication for Enhanced Security
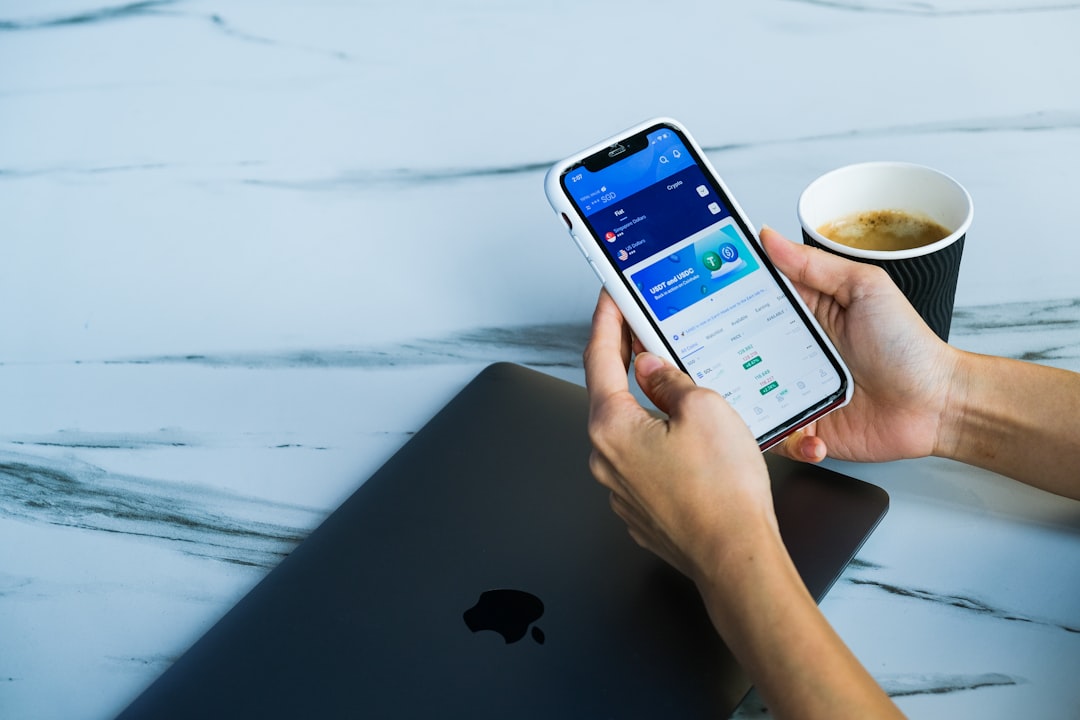
When building a Python-based trading bot, especially one that interacts with platforms like Robinhood, security should be a top priority. Implementing multi-factor authentication (MFA) significantly enhances security by requiring users to provide multiple forms of verification before granting access. This can be achieved using Python libraries like `pyotp` to manage Time-Based One-Time Passwords (TOTP), a common form of two-factor authentication (2FA).
The process typically involves the bot generating a unique secret key for each user, which is then used to create and validate OTPs. Users can conveniently handle these OTPs via apps like Google Authenticator, easily provisioning new OTP secrets with QR codes. However, implementing MFA requires careful consideration. Thorough testing is essential to ensure the security mechanisms are reliable and effective, given the constantly evolving landscape of online threats.
By adding this additional layer of security, you significantly reduce the risk of unauthorized access to trading bots and the financial data they handle. While it's a critical step, MFA alone isn't a complete solution. It's important to understand its limitations and potentially combine it with other security best practices for a more comprehensive approach.
Implementing multi-factor authentication (MFA) is a critical step in beefing up security, especially for applications handling sensitive information like trading bots. Research suggests that MFA can drastically reduce the chances of unauthorized access, making it a valuable defense against malicious actors.
MFA works by demanding two or more verification steps, like a password and a code from an authenticator app. This layered approach creates a tougher barrier against intruders, making it significantly harder to gain access to accounts compared to just using a password.
While SMS-based MFA is a common choice, it's important to understand that it’s more susceptible to security flaws, such as SIM-swapping attacks. App-based or hardware tokens generally offer stronger protection.
Interestingly, despite the strong security benefits, the adoption rate of MFA is relatively low across user bases. This suggests a knowledge gap about its importance, which could be addressed with better educational efforts.
It's also worth noting that implementing MFA can sometimes be a source of friction for users. The extra steps and management of multiple authentication methods can be a hassle, which could cause users to adopt less secure habits to bypass the hassle.
Further, integrating MFA into automated trading systems presents some unique challenges. The delay introduced by MFA verification steps might slow down the responsiveness of bots that need quick access to market data, which requires a trade-off between security and performance.
This responsiveness concern is more pronounced with systems like those designed to work with the Robinhood API, which often necessitate rapid execution of trades. Keeping MFA tokens fresh and managing session expiry are key concerns in designing an MFA implementation within a bot's logic.
Over time, users can get accustomed to security protocols, a phenomenon called security fatigue. This complacency could lead to users actively avoiding or becoming less careful with MFA steps. Counteracting this requires proactive strategies to maintain user engagement with security protocols.
It's also important to acknowledge that compliance with regulations often mandates MFA for applications dealing with financial data and personal information. This adds another layer of responsibility in designing any trading system built on top of the Robinhood API or similar platforms, making it essential to design the entire system with both functionality and compliance in mind.
Finally, there's still ongoing research and development into MFA, with areas like machine learning and biometrics potentially enhancing the capabilities and security of existing approaches in the future.
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Analyzing Market Data and Generating Automated Trading Signals
1. The idea that automated trading systems can potentially outperform human traders is intriguing. By rapidly analyzing massive amounts of market data, these systems can theoretically make better decisions and avoid the emotional biases that often cloud human judgment in trading. It's a compelling proposition, but there are important caveats.
2. Machine learning has shown promise in boosting the quality of trading signals. These algorithms can uncover patterns in historical data that might not be evident using traditional technical indicators, potentially leading to more accurate predictions of future market moves. However, it's crucial to remember that these models are only as good as the data they're trained on.
3. The volume of data analyzed seems to play a significant role in the effectiveness of automated trading. More data usually leads to more statistically robust results. For example, bots that rely on high-frequency trading data often have a more solid foundation for generating signals than those working with smaller datasets. This suggests that data availability and quality might be a limiting factor.
4. Automated strategies can introduce hidden risks like "factor exposures". These can arise from over-reliance on certain technical indicators, which can lead to unexpected behavior in the market during volatile periods. This is a critical issue as it demonstrates that even carefully designed trading strategies can have unforeseen consequences.
5. In the realm of automated trading, the time it takes between when a trading signal is generated and when it's executed can have a major impact on a trader's success. Even very small delays, like a few milliseconds, can affect buy and sell decisions. This highlights the need for trading bots to be built on very low-latency infrastructure.
6. While the Robinhood API is useful for getting access to trading data, it does have some drawbacks, such as rate limits and potentially delayed data updates. These limitations can make building effective trading strategies challenging. I wonder if we can find innovative solutions like caching data locally or using alternative data sources to address these issues.
7. Instead of just focusing on price movements, volume profile indicators can give us a deeper understanding of the market's liquidity. This helps in recognizing potential support and resistance levels which could contribute to more precise trading signals. It's an interesting approach that adds another layer to market analysis.
8. Most trading strategies need to be consistently evaluated and changed to adapt to how the market's behaving. This means a trading signal algorithm that performed well in the past might not be suitable for current conditions, highlighting the importance of being agile.
9. The accuracy of the data that goes into automated trading decisions is incredibly important. If the data is inaccurate, either because of unreliable feeds or incomplete historical data, it can lead to signals that aren't trustworthy, which ultimately impacts trading results. This seems like a major concern for any algorithmic trading system.
10. It's interesting that relying on automated systems might cause traders to become less vigilant in monitoring their strategies. It's crucial to acknowledge this potential psychological impact and find ways to balance automation with ongoing human oversight. It's a bit of a human factor issue we might not immediately think about with these systems.
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Incorporating Cryptocurrency Trading with Robinhood's Crypto Wallet Integration
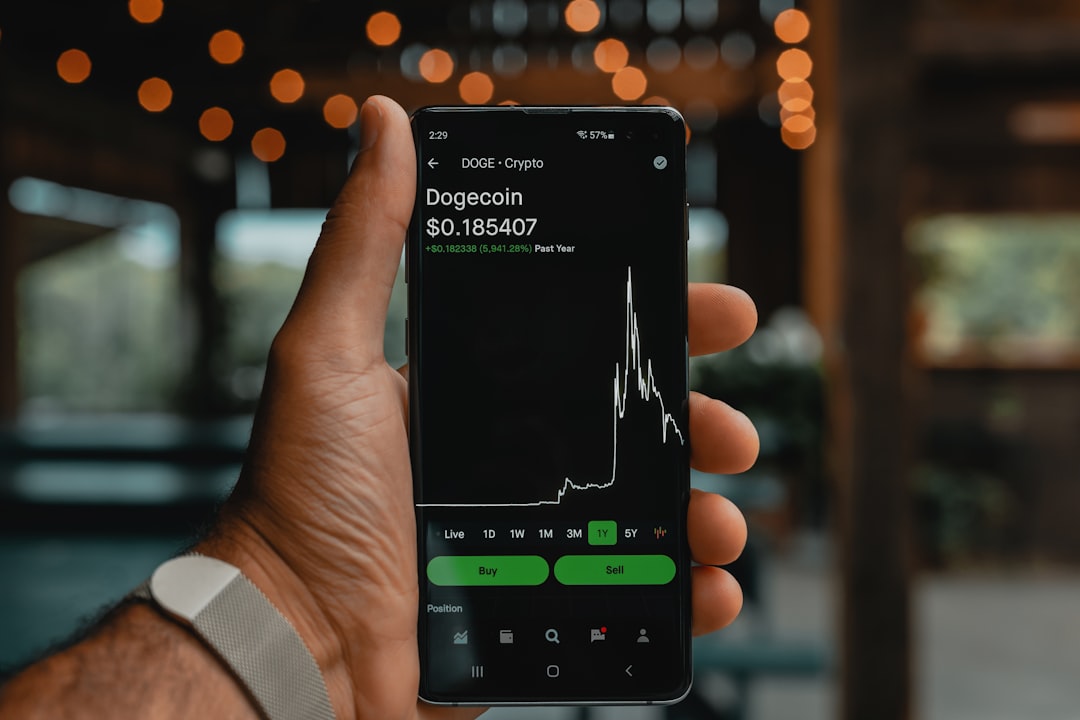
Python-Based Trading Bot for Robinhood A Deep Dive into Automated Strategies and API Integration - Leveraging Twitter API for Sentiment Analysis in Trading Strategies
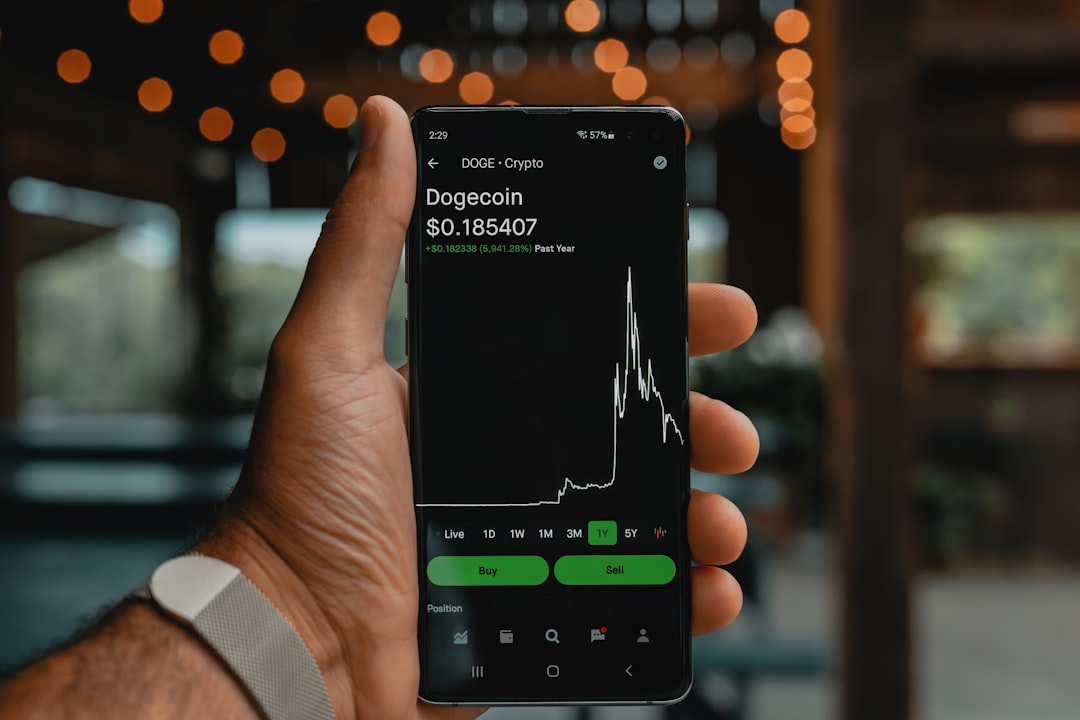
Integrating the Twitter API into trading strategies offers a fresh way to incorporate public sentiment into algorithmic trading decisions. We can harness natural language processing to analyze real-time Twitter conversations related to particular stocks, turning social media chatter into potentially valuable trading signals. Python libraries like Tweepy and VADER are often used for this, making it possible to incorporate Twitter sentiment into trading bots and develop more complex decision-making algorithms.
However, it's essential to consider that sentiment expressed on Twitter is often fleeting and doesn't always directly translate into tangible market actions. Relying solely on Twitter-based sentiment for trading can be risky. A smart approach would be to balance it with traditional technical analysis and a thorough understanding of market fundamentals. This way, we can develop trading strategies that are more informed and potentially more resilient in the face of unpredictable market conditions.
The Twitter API offers a way to tap into a huge pool of data, including individual tweets, user profiles, and how people interact, which is important for figuring out how people feel about the market. By understanding this sentiment, traders might get a sense of the public's opinion on specific stocks or market conditions before making their trades.
Some research suggests that the way people talk about stocks on social media might sometimes be able to predict how stock prices will change, sometimes even before traditional financial indicators show anything. This suggests that the growing number of retail investors who actively discuss their trades on platforms like Twitter can have a real impact on the market.
Using natural language processing, or NLP, traders can analyze the sentiment of a large number of tweets and classify them as positive, negative, or neutral. Tools like VADER, built specifically for sentiment analysis, can provide seemingly accurate results by focusing on the context of financial conversations.
There's a bit of a delay between when people express a positive outlook on Twitter and when the price of a stock actually goes up. For example, if a lot of people are saying positive things about a company, it tends to lead to a price increase, but not immediately. Knowing this time delay is vital for traders to get the best results using sentiment-based strategies.
Bots using the Twitter API can process sentiment information in real-time. This allows trading systems that react quickly to changes in public opinion, a benefit particularly in situations where markets are volatile and fast decisions are essential. This speed can help them stay ahead of the game.
The sheer volume of data on Twitter could be a fantastic resource for machine learning models that are trying to predict stock movements. However, Twitter data can be very noisy and sometimes unreliable, so it's important to be cautious about bias in the models.
Interestingly, looking at massive sets of Twitter data suggests that tweets with informal language or slang might be more strongly connected to real market changes compared to formal business language. This implies that traders might need to adapt their sentiment analysis tools to accurately capture the nuances of online conversations.
A challenge with using the Twitter API for sentiment analysis is making sure the data you're looking at is truly representative of the market. Just because there's a big increase in the number of tweets about a stock doesn't mean it's a meaningful signal. So, filtering out the truly valuable information from all the noise is a big factor for traders to consider.
Engaging with Twitter in real-time does carry some risk, like traders being influenced by quickly spreading emotional reactions rather than focusing on the basics of the company. This psychological element means that trading strategies need to be designed with discipline when they incorporate social sentiment.
Lastly, combining the insights from Twitter sentiment analysis with traditional ways of looking at the market can make trading strategies much stronger and lead to more informed decisions. This blended approach of fundamental analysis and crowd psychology may result in better prediction outcomes.
More Posts from :